I did just have an idea on how to do this…
create a filter to correct the colors.
What is a color filter? Lets look at it from the standpoint of using an old school film camera. A filter is simply a lens that screws onto the camera. The lens is simply a colored piece of glass. Do the same thing but digitally. Create an object that has the same width and height as the screen. Remove all borders, outlines, padding and margins. Make the object transparent to input using the flags and then set all colors to completely transparent for all of the different pieces like the border, shadows and outlines. Now you can set the color for the background and set the opacity so it acts like a filter. I do wish that LVGL had a way of setting a blend mode so all objects behind it would be blended as an overlay instead of additive.
@kisvegabor would that be a feature that you would have interest in adding?
The first solution I gave in my previous post might actually be the better one because you can use an overlay algorithm to blend the colors. overlay blending is not additive. take this as an example. If you could take a color sample of what is being seen by a set of eyes from a display and the color what is being seen is 245, 255, 255 and you want it to be 255, 255, 255 you use overlay blending and place a color of 255, 245, 245 over the top of it. the resulting color ends up being 255, 255, 255. where as with a normal type blending you would need to mess with the alpha channel and that could end up causing an uneven distribution of the correction across the image. with overlay blending you set the alpha to 255 and leave it.
Here is an example.
You have this color which is 20, 113, 35
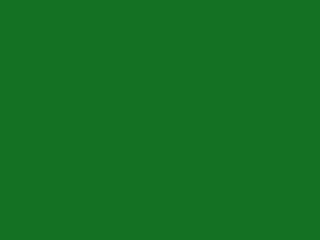
and you overlay it with 101, 55, 255
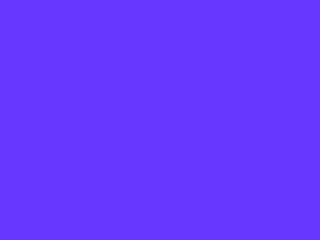
the resulting color is 16, 49, 70
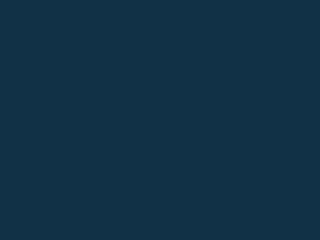
here is an example written in Python.
pixel = [20, 113, 35]
overlay = [101, 55, 255]
def overlay_blend(base_c, overlay_c):
if base_c > 127:
result = int(round(overlay_c * ((255 - base_c) / 127) + (base_c - (255 - base_c))))
else:
result = int(round(overlay_c * (base_c / 127)))
return result
for i in range(3):
pixel[i] = overlay_blend(pixel[i], overlay[i])
print(pixel)
# output is [16, 49, 70]